Query Parameters
One of the most used features is the ability to pass query parameters to the SDK as URL Search Params (query params). This allows you to customize the SDK behavior and use your Living App, for example, in different environments while specifying the backend URL.
Since the SDK will be included in the Living App as a <script>
, we are using these URL Search Params as a hot load configuration for the Living Apps.
These query parameters are used by the devTools as well:
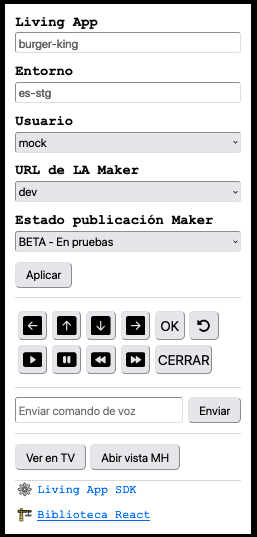
?mock
true
, false
, aura
.
On document load, the SDK will start the Living App initialization process: register the listeners, set the splash page, read the config, connect to the backend, connect to external internal services (Aura), etc.
Mock value is determined by these rules:
- Top-most priority: if the env var
LA_CORE_I_AM_NOT_A_LIVING_APP
is on, mock will be true. - Query param
?mock
. - Defaults:
- GVP (Brazil): true (use query param
?mock=aura
if you need to override this default). - localhost: true.
- GVP (Brazil): true (use query param
- Otherwise, mock is false.
What does it mean to mock the SDK?
When mock mode is enabled...
- These modules are replaced:
Module | Desc. | Mock replacement |
---|---|---|
Internal Authentication | Get userId and accessToken on initialization | Mocked userId and accessToken values |
Aura Client | Dialog Context features | Mimic using raw memory |
Storage Client | Equivalent to local storage (on the LAB) | window.localStorage |
Terms & Conds. | T&C mandatory page | Dummy behavior: Terms are accepted |
- These modules have some behavior mocked:
Module | Desc. | Mock Behavior |
---|---|---|
HTTP Client | General purpose HTTP request through the LAB | No Bearer authorization header |
Telefonica API Client | HTTP requests to Telefonica Kernel service | Login redirect URI |
- These modules read the mock status:
Module | Desc. | Read mock status |
---|---|---|
Metrics Client | App Insights client | Initialization telemetry item: data.mockEnabled |
Mocking the Internal Authentication on initialization
When the mock is enabled, the SDK will not try to authenticate the user. This means that the SDK will not try to get the access_token
from the LAB DB.
userId = 'mock-userId';
accessToken = 'mock-accesstoken';
Mocking the Aura Client module
The following exposed methods are calling directly the Aura Client module:
laSdk.getDialogContext()
laSdk.setDialogContext()
laSdk.addToDialogContext()
laSdk.removeFromDialogContext()
laSdk.clearDialogContext()
laSdk.sendText()
When the mock is enabled (?mock=true
or ?mock=aura
), the SDK will use a mocked implementation of the Aura Client module which mimics the behavior using raw memory.
const persistence: Record<string, string> = {};
const dialogContext: { text: string; event: string }[] = [];
Mocking the Storage module
The following exposed methods are calling directly the Storage module:
laSdk.persistenceDrop()
laSdk.persistenceWrite(key, value)
laSdk.persistenceRead(key)
When the mock is enabled, the SDK will use regular window.localStorage
to store the data instead of the LAB Storage module.
By default, the SDK will use the LAB DB to store the data:
laSdk.persistenceWrite('foo', '42')
// -> POST /utils/storage { key: 'foo', value: '42', living_app_name }
laSdk.persistenceRead('foo')
// -> GET /utils/storage?living_app_name
Mocking the Terms & Conditions module
If mock is enabled, the SDK will not show the T&C mandatory page.
Mocking the HTTP Client
Unless the mock is enabled, the HTTP client will include the Bearer authorization
header. If mock is enabled, the HTTP client will not include the authorization
header.
laSdk.http.get()
laSdk.http.post()
laSdk.http.put()
laSdk.http.patch()
laSdk.http.delete()
Unlike other modules, the HTTP client will not be replaced by a mock implementation. The HTTP client will work as usual, but the authorization
header will not be included.
Mocking the Telefonica API Client
on laSdk.telefonicaAPI.login()
: the redirect URI will have the query parameter ?authorization_id=mockLOA3
.
Unlike other modules, the Telefonica API Client client will not be replaced by a mock implementation. The client will work as usual, but the login()
method will have a mocked redirect URI.
Mocking the Metrics Client
Initialization telemetry item includes data.mockEnabled
?env
es-stg
, es-pre
, es-pro
, br-srg
, br-pre
, br-pro
.
The environment where the Living App is running. This value is read by HTTP clients and other services to apply different configurations.
By default the SDK will infer the environment from the window.location.host
, but you can override it using this query parameter. e.g. ?env=es-stg
.
Other configurations that will read the environment: dev-tools, SDF client, App Insights, internal metrics...
?labUrl
URL of the backend API such as https://api.dev.livingappsmaker.com/
, https://api.beta.livingappsmaker.com/
...
URL of the Backend API.
By default, the SDK will use the URL inferred from the environment (which, in turn, depends reads window.location.host
by default), but you can override it using this query parameter, setting explicitly the URL of the backend API.
lab stands for: Living Apps Backend
Existing backend URLs (clicking in the links will show the deployed API version and list the running services):
?labUrl | Description |
---|---|
https://api.dev.livingappsmaker.com/ | Contains previous release |
https://api-livingappsmaker.cdncert.telefonica.com | TCDN for dev |
https://api.beta.livingappsmaker.com/ | Continuous deployment of master branch |
https://api.es-qa.livingappsmaker.com/ | Testing for ES |
https://api.es-pre.livingappsmaker.com/ | ES PRE (OB owner) |
https://api.es-pro.livingappsmaker.com/ | ES PRO (OB owner) |
https://api-livingappsmaker.cdn.telefonica.com | CDN for ES PRO |
https://api.br-qa.livingappsmaker.com/ | Testing for BR |
https://api.br-pre.livingappsmaker.com/ | BR PRE (OB owner) |
https://api.br-pro.livingappsmaker.com/ | BR PRO (OB owner) |
Working with the HTTP clients.
laSdk.telefonicaApi.get('/api/foo')
// -> GET ${labUrl}/telefonica-kernel/api/foo
?contentsLabEnv
pre
, pro
.
Translates to the URL where the content - screens, resources and settings - of the Living App are published.
By default it is set to pro
(live) unless the env
is defined as 'es-pre'
; but you can override it using this query parameter
?contentsLabEnv | blob storage URL |
---|---|
pre | (blob URL)/maker/contents/{laName}/beta/ |
pro (default) | (blob URL)/maker/contents/{laName}/live/ |
Content Creation in the Living Apps Maker:
Living Apps Maker is the tool used to create generic Living Apps.
Each environment (dev
, beta
, es-qa
, es-pre
, es-pro
, br-qa
, br-pre
, br-pro
) has its own instance of the Maker.
These instances are isolated from each other, meaning that the content creator user works in an specific environment.
When creating a Living App in the Living Apps Maker, the user creates content:
- layouts (the screens)
- resources (e.g. the splash page)
- settings
this content is always updating in real time in the DB of the specific environment. The content is in draft
mode.
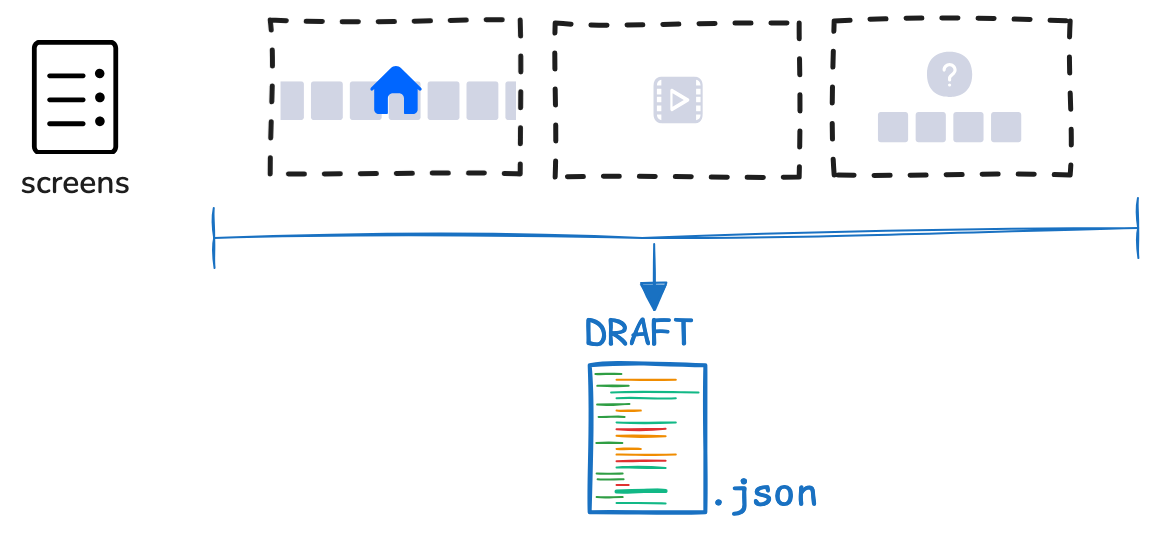
To publish these contents to the Azure Blob storage (remember, each instance of the Maker points to its own Blob storage), the user has to publish the content.
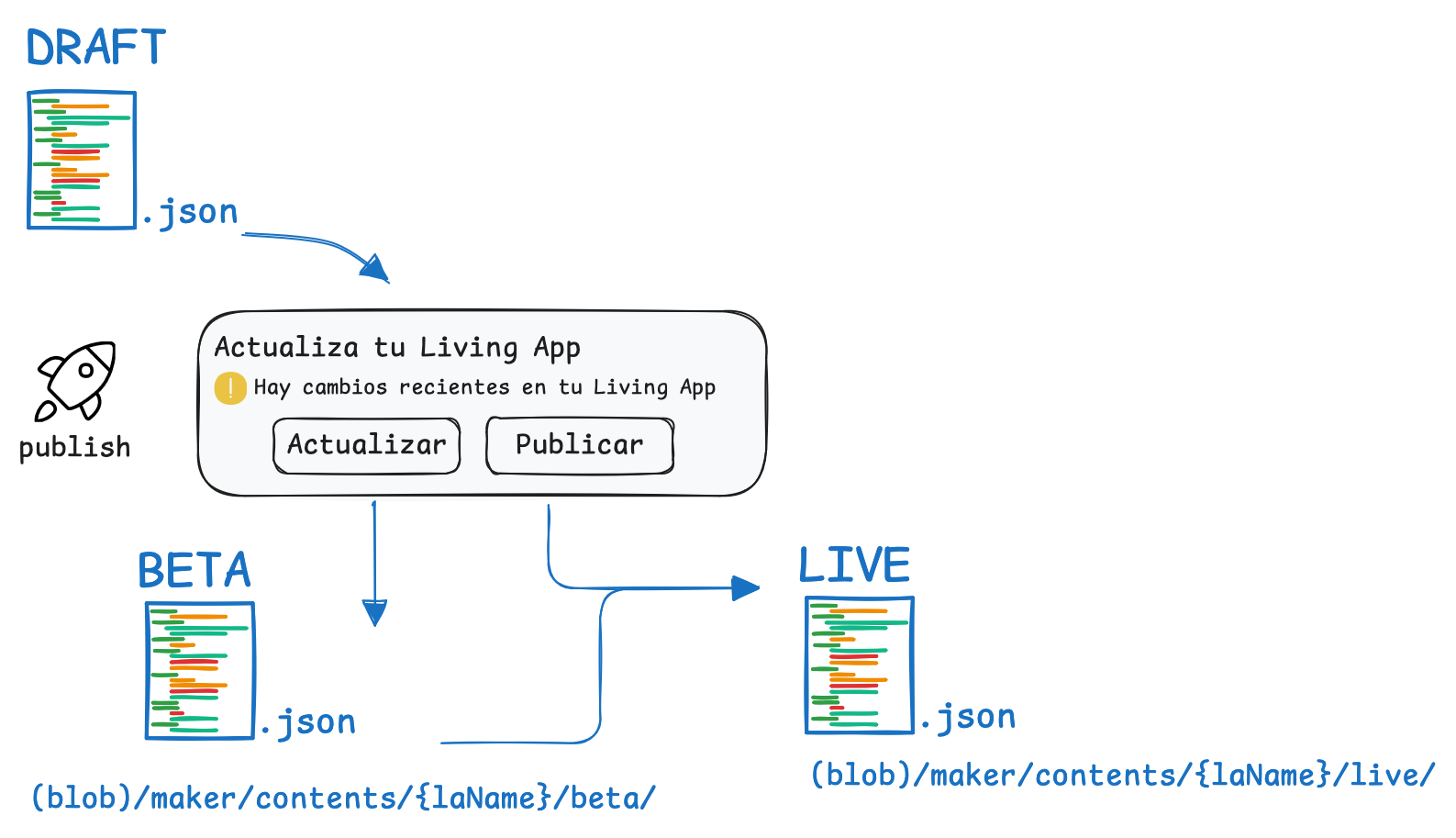
Contents can be published in two different "stages": beta
(formerly called pre
) and live
(formerly called pro
).
There are three possible stages for the content created in the Maker:
draft
: the content is being edited in real time.beta
: the content is published to the Blob storage/maker/contents/{laName}/beta/
live
: the content is published to the Blob storage/maker/contents/{laName}/live/
Note: do NOT confuse the environment named beta
with the beta
stage of the contents.
?contentsLabUrl
URL of the Blob storage such as https://lamakersabeta.blob.core.windows.net/
,
https://lamakersaesqa.blob.core.windows.net/
...
Defines the Blob URL where the screens are published.
By default this is the same as the labUrl
, but you can override it setting explicitly a different LAB URL which is translated to the Blob URL where the screens are published.
?contentsLabUrl | Storage Account | Blob URL |
---|---|---|
https://api.beta.livingappsmaker.com (beta ) | lamakersabeta | https://lamakersabeta.blob.core.windows.net |
https://api.es-qa.livingappsmaker.com (es-qa ) | lamakersaesqa | https://lamakersaesqa.blob.core.windows.net |
https://api.es-pre.livingappsmaker.com (es-pre ) | lamakersaespre | https://lamakersaespre.blob.core.windows.net |
https://api.es-pro.livingappsmaker.com (es-pro ) | lamakersaespro | https://lamakersaespro.blob.core.windows.net |
https://api.br-qa.livingappsmaker.com (br-qa ) | lamakersabrqa | https://lamakersabrqa.blob.core.windows.net |
https://api.br-pre.livingappsmaker.com (br-pre ) | lamakersabrpre | https://lamakersabrpre.blob.core.windows.net |
https://api.br-pro.livingappsmaker.com (br-pro ) | lamakersabrpro | https://lamakersabrpro.blob.core.windows.net |
https://api.dev.livingappsmaker.com (es-stg ) | lamakersadev | https://lamakersadev.blob.core.windows.net |
Note: There is an escape hatch to use exactly the same URL for both the LAB and the Blob, ending the
labUrl
with the ports:8889
or:8091
(e.g.?labUrl=localhost:8889/
). The list of allowed ports can be found in this file:src/utils/config.ts
.
Working with the Maker Contents client
Example: if ?contentsLabUrl
is https://api.beta.livingappsmaker.com/
, and considering that contentsLabEnv
has the default value (not specifically on 'es-pre'
, neither overriding the ?contentsLabEnv
parameter),
the SDK will use the following URLs to fetch the content (screens) created in the Maker:
laSdk.getMakerContentsHome()
// -> GET https://lamakersabeta.blob.core.windows.net\
// /maker/contents/{laName}/live/home_layout.json
laSdk.getAllLayouts()
// -> GET https://lamakersabeta.blob.core.windows.net\
// /maker/contents/{laName}/live/all_layouts.json
laSdk.getMakerContents('foo')
// -> GET https://lamakersabeta.blob.core.windows.net\
// /maker/contents/{laName}/live/all_layouts.json
// .find(layout.id === 'foo')
?laName
string
Living Apps created by the Living Apps Maker are identified by a unique name. This query parameter allows us to specify which generic Living App to load.
?splash_url
string
The Living App will render a splash image (1920x1080 static image) during initialization.
By default, the splash image URL is constructed using the ?contentsLabUrl
and ?laName
values, following this pattern:
`${?contentsLabUrl}/maker/contents/${?laName}/${live | beta}/resources/stb_splash`
For example, for the es-pro
environment:
https://lamakersaespro.blob.core.windows.net/maker/contents/{laName}/live/resources/stb_splash
You can override the default splash image URL by providing the ?splash_url
query parameter. This is useful for testing or changing the splash image between redirections.
?browser
Deprecated
?sessionId
Deprecated
?dChannel
Deprecated
?weinre
true
Setting ?weinre=true
enables the remote web inspector - Weinre - for debugging on STB. Equivalent to press «8
» for 10 seconds. Access https://weinre.apps.ocp-epg.tid.es/